Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
match/app/config/routes.php
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
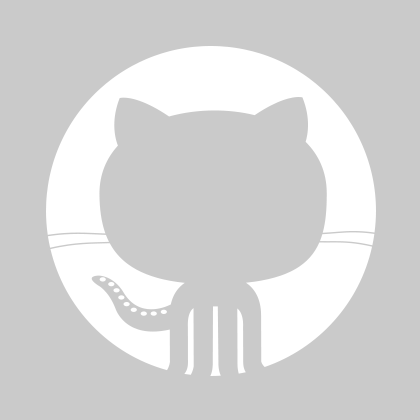
…ntroller (CO-2470)
107 lines (99 sloc)
4.09 KB
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
/** | |
* Routes configuration. | |
* | |
* In this file, you set up routes to your controllers and their actions. | |
* Routes are very important mechanism that allows you to freely connect | |
* different URLs to chosen controllers and their actions (functions). | |
* | |
* It's loaded within the context of `Application::routes()` method which | |
* receives a `RouteBuilder` instance `$routes` as method argument. | |
* | |
* CakePHP(tm) : Rapid Development Framework (https://cakephp.org) | |
* Copyright (c) Cake Software Foundation, Inc. (https://cakefoundation.org) | |
* | |
* Licensed under The MIT License | |
* For full copyright and license information, please see the LICENSE.txt | |
* Redistributions of files must retain the above copyright notice. | |
* | |
* @copyright Copyright (c) Cake Software Foundation, Inc. (https://cakefoundation.org) | |
* @link https://cakephp.org CakePHP(tm) Project | |
* @license https://opensource.org/licenses/mit-license.php MIT License | |
*/ | |
use Cake\Routing\Route\DashedRoute; | |
use Cake\Routing\RouteBuilder; | |
/* | |
* The default class to use for all routes | |
* | |
* The following route classes are supplied with CakePHP and are appropriate | |
* to set as the default: | |
* | |
* - Route | |
* - InflectedRoute | |
* - DashedRoute | |
* | |
* If no call is made to `Router::defaultRouteClass()`, the class used is | |
* `Route` (`Cake\Routing\Route\Route`) | |
* | |
* Note that `Route` does not do any inflections on URLs which will result in | |
* inconsistently cased URLs when used with `:plugin`, `:controller` and | |
* `:action` markers. | |
*/ | |
/** @var \Cake\Routing\RouteBuilder $routes */ | |
$routes->setRouteClass(DashedRoute::class); | |
$routes->scope('/', function (RouteBuilder $builder) { | |
/* | |
* Here, we are connecting '/' (base path) to a controller called 'Pages', | |
* its action called 'display', and we pass a param to select the view file | |
* to use (in this case, templates/Pages/home.php)... | |
*/ | |
$builder->connect('/', ['controller' => 'Pages', 'action' => 'display', 'home']); | |
/* | |
* ...and connect the rest of 'Pages' controller's URLs. | |
*/ | |
$builder->connect('/pages/*', 'Pages::display'); | |
/** | |
* TAP ID Match API Routes | |
*/ | |
// Match Request | |
$builder->get('/api/{matchgrid_id}/v1/matchRequests/{id}', ['controller' => 'TapApi', 'action' => 'viewMatchRequest']); | |
$builder->get('/api/{matchgrid_id}/v1/matchRequests', ['controller' => 'TapApi', 'action' => 'viewMatchRequests']); | |
$builder->put('/api/{matchgrid_id}/v1/people/{sor}/{sorid}', ['controller' => 'TapApi', 'action' => 'match']); | |
$builder->post('/api/{matchgrid_id}/v1/people/{sor}/{sorid}', ['controller' => 'TapApi', 'action' => 'search']); | |
// This doesn't match and we end up in current(), so we just check there | |
// Also, as of API v1.0.0, search over GET has been removed | |
// $builder->get('/api/{matchgrid_id}/v1/people/{sor}/{sorid}?*', ['controller' => 'TapApi', 'action' => 'search']); | |
$builder->delete('/api/{matchgrid_id}/v1/people/{sor}/{sorid}', ['controller' => 'TapApi', 'action' => 'remove']); | |
$builder->get('/api/{matchgrid_id}/v1/people/{sor}/{sorid}', ['controller' => 'TapApi', 'action' => 'current']); | |
$builder->get('/api/{matchgrid_id}/v1/people/{sor}', ['controller' => 'TapApi', 'action' => 'inventory']); | |
$builder->put('/api/{matchgrid_id}/v1/referenceIds/{id}', ['controller' => 'TapApi', 'action' => 'merge']); | |
/** | |
* Connect catchall routes for all controllers. | |
* | |
* The `fallbacks` method is a shortcut for | |
* | |
* ``` | |
* $builder->connect('/{controller}', ['action' => 'index']); | |
* $builder->connect('/{controller}/{action}/*', []); | |
* ``` | |
* | |
* You can remove these routes once you've connected the | |
* routes you want in your application. | |
*/ | |
$builder->fallbacks(); | |
}); | |
/* | |
* If you need a different set of middleware or none at all, | |
* open new scope and define routes there. | |
* | |
* ``` | |
* $routes->scope('/api', function (RouteBuilder $builder) { | |
* // No $builder->applyMiddleware() here. | |
* | |
* // Parse specified extensions from URLs | |
* // $builder->setExtensions(['json', 'xml']); | |
* | |
* // Connect API actions here. | |
* }); | |
* ``` | |
*/ |