Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
match/app/src/Application.php
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
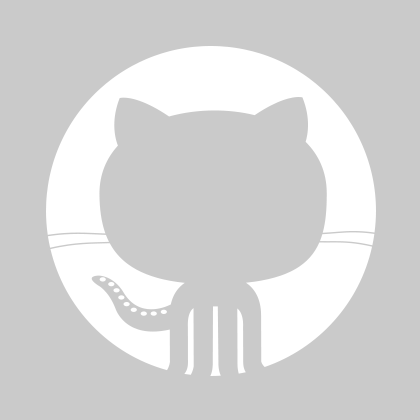
…ntroller (CO-2470)
84 lines (74 sloc)
3.18 KB
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
/** | |
* CakePHP(tm) : Rapid Development Framework (https://cakephp.org) | |
* Copyright (c) Cake Software Foundation, Inc. (https://cakefoundation.org) | |
* | |
* Licensed under The MIT License | |
* For full copyright and license information, please see the LICENSE.txt | |
* Redistributions of files must retain the above copyright notice. | |
* | |
* @copyright Copyright (c) Cake Software Foundation, Inc. (https://cakefoundation.org) | |
* @link https://cakephp.org CakePHP(tm) Project | |
* @since 3.3.0 | |
* @license https://opensource.org/licenses/mit-license.php MIT License | |
*/ | |
namespace App; | |
use Cake\Error\Middleware\ErrorHandlerMiddleware; | |
use Cake\Http\BaseApplication; | |
use Cake\Http\Middleware\CsrfProtectionMiddleware; | |
use Cake\Http\Middleware\BodyParserMiddleware; | |
use Cake\Routing\Middleware\AssetMiddleware; | |
use Cake\Routing\Middleware\RoutingMiddleware; | |
use Psr\Http\Message\ServerRequestInterface; | |
/** | |
* Application setup class. | |
* | |
* This defines the bootstrapping logic and middleware layers you | |
* want to use in your application. | |
*/ | |
class Application extends BaseApplication { | |
/** | |
* Setup the middleware queue your application will use. | |
* | |
* @param \Cake\Http\MiddlewareQueue $middlewareQueue The middleware queue to setup. | |
* @return \Cake\Http\MiddlewareQueue The updated middleware queue. | |
*/ | |
public function middleware(\Cake\Http\MiddlewareQueue $middlewareQueue): \Cake\Http\MiddlewareQueue { | |
// loading csrf Middleware | |
$csrf = new CsrfProtectionMiddleware(['cookieName' => 'matchCsrfToken']); | |
$csrf->skipCheckCallback(function (ServerRequestInterface $request) { | |
// skip controllers | |
$skipedControllers = ['TapApi']; // Controllers list | |
if(in_array($request->getParam('controller'), $skipedControllers, true)) { | |
return true; | |
} | |
// skip debugkit | |
if($request->getParam('plugin') === 'DebugKit') { | |
return true; | |
} | |
return $request; | |
}); | |
$middlewareQueue | |
// Catch any exceptions in the lower layers, | |
// and make an error page/response | |
->add(ErrorHandlerMiddleware::class) | |
// Handle plugin/theme assets like CakePHP normally does. | |
->add(AssetMiddleware::class) | |
// Add routing middleware. | |
->add(new RoutingMiddleware($this)) | |
// https://book.cakephp.org/4/en/controllers/middleware.html#body-parser-middleware | |
// Enable requests decoding into an array that is available via $request->getParsedData() | |
// and $request->getData() | |
// // only JSON will be parsed | |
->add(new BodyParserMiddleware()) | |
// Enable CSRF protection using Cake v3.5+ approach. | |
// Initially, we use the default options, except a different CSRF cookie | |
// name to avoid conflicts with Registry. Additionally, we don't want CSRF | |
// checking enabled on API requests (which are stateless and should not be | |
// called from web browsers). See eg | |
// https://stackoverflow.com/questions/47714940/cakephp-3-5-6-disable-csrf-middleware-for-controller | |
// https://stackoverflow.com/questions/51931406/post-requests-for-cakephp-3-api-are-not-working | |
->add($csrf); | |
return $middlewareQueue; | |
} | |
} |