Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
codeql-action/node_modules/lodash/_compareMultiple.js
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
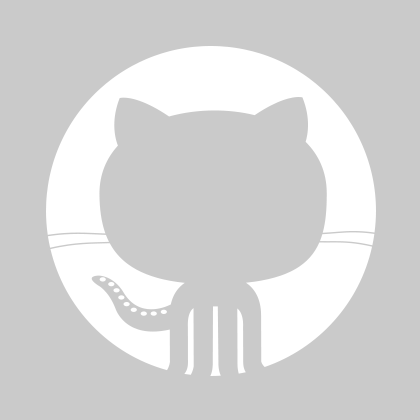
44 lines (41 sloc)
1.56 KB
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
var compareAscending = require('./_compareAscending'); | |
/** | |
* Used by `_.orderBy` to compare multiple properties of a value to another | |
* and stable sort them. | |
* | |
* If `orders` is unspecified, all values are sorted in ascending order. Otherwise, | |
* specify an order of "desc" for descending or "asc" for ascending sort order | |
* of corresponding values. | |
* | |
* @private | |
* @param {Object} object The object to compare. | |
* @param {Object} other The other object to compare. | |
* @param {boolean[]|string[]} orders The order to sort by for each property. | |
* @returns {number} Returns the sort order indicator for `object`. | |
*/ | |
function compareMultiple(object, other, orders) { | |
var index = -1, | |
objCriteria = object.criteria, | |
othCriteria = other.criteria, | |
length = objCriteria.length, | |
ordersLength = orders.length; | |
while (++index < length) { | |
var result = compareAscending(objCriteria[index], othCriteria[index]); | |
if (result) { | |
if (index >= ordersLength) { | |
return result; | |
} | |
var order = orders[index]; | |
return result * (order == 'desc' ? -1 : 1); | |
} | |
} | |
// Fixes an `Array#sort` bug in the JS engine embedded in Adobe applications | |
// that causes it, under certain circumstances, to provide the same value for | |
// `object` and `other`. See https://github.com/jashkenas/underscore/pull/1247 | |
// for more details. | |
// | |
// This also ensures a stable sort in V8 and other engines. | |
// See https://bugs.chromium.org/p/v8/issues/detail?id=90 for more details. | |
return object.index - other.index; | |
} | |
module.exports = compareMultiple; |