Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
codeql-action/node_modules/typed-rest-client/Util.js
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
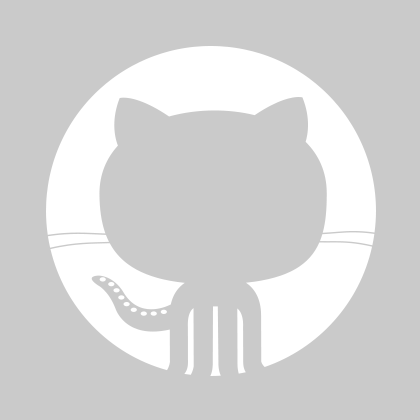
35 lines (35 sloc)
1.35 KB
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
"use strict"; | |
// Copyright (c) Microsoft. All rights reserved. | |
// Licensed under the MIT license. See LICENSE file in the project root for full license information. | |
Object.defineProperty(exports, "__esModule", { value: true }); | |
const url = require("url"); | |
const path = require("path"); | |
/** | |
* creates an url from a request url and optional base url (http://server:8080) | |
* @param {string} resource - a fully qualified url or relative path | |
* @param {string} baseUrl - an optional baseUrl (http://server:8080) | |
* @return {string} - resultant url | |
*/ | |
function getUrl(resource, baseUrl) { | |
const pathApi = path.posix || path; | |
if (!baseUrl) { | |
return resource; | |
} | |
else if (!resource) { | |
return baseUrl; | |
} | |
else { | |
const base = url.parse(baseUrl); | |
const resultantUrl = url.parse(resource); | |
// resource (specific per request) elements take priority | |
resultantUrl.protocol = resultantUrl.protocol || base.protocol; | |
resultantUrl.auth = resultantUrl.auth || base.auth; | |
resultantUrl.host = resultantUrl.host || base.host; | |
resultantUrl.pathname = pathApi.resolve(base.pathname, resultantUrl.pathname); | |
if (!resultantUrl.pathname.endsWith('/') && resource.endsWith('/')) { | |
resultantUrl.pathname += '/'; | |
} | |
return url.format(resultantUrl); | |
} | |
} | |
exports.getUrl = getUrl; |