Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
codeql-action/lib/util.test.js
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
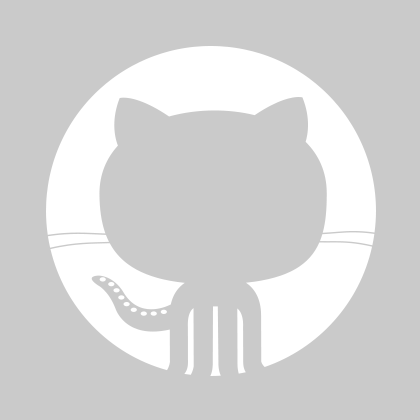
Andrew Eisenberg
Allow the codeql-action to be run locally (#117)
* Allow the codeql-action to be run locally This change allows the codeql-action to be run locally through [act](https://github.com/nektos/act). In order to run the action locally, you need to do two things: 1. Add the `CODEQL_LOCAL_RUN: true` environment variable. The only way I could figure out how to do this was to add it directly in the workflow file in an `env` block. It _should_ be possible to add it through a `.env` file and pass it to `act`, but I couldn't get it working. 2. Run this command `act -j codeql -s GITHUB_TOKEN=<MY_PAT>` Setting the `CODEQL_LOCAL_RUN` env var will fill in missing env vars that the action needs, but isn't set by `act`. It will also avoid making api calls to github that would fail locally. This is a refactoring discussed in https://github.com/github/dsp-codeql/issues/36
95 lines (95 sloc)
3.61 KB
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
"use strict"; | |
var __importDefault = (this && this.__importDefault) || function (mod) { | |
return (mod && mod.__esModule) ? mod : { "default": mod }; | |
}; | |
var __importStar = (this && this.__importStar) || function (mod) { | |
if (mod && mod.__esModule) return mod; | |
var result = {}; | |
if (mod != null) for (var k in mod) if (Object.hasOwnProperty.call(mod, k)) result[k] = mod[k]; | |
result["default"] = mod; | |
return result; | |
}; | |
Object.defineProperty(exports, "__esModule", { value: true }); | |
const ava_1 = __importDefault(require("ava")); | |
const fs = __importStar(require("fs")); | |
const os = __importStar(require("os")); | |
const testing_utils_1 = require("./testing-utils"); | |
const util = __importStar(require("./util")); | |
testing_utils_1.setupTests(ava_1.default); | |
ava_1.default('getToolNames', t => { | |
const input = fs.readFileSync(__dirname + '/../src/testdata/tool-names.sarif', 'utf8'); | |
const toolNames = util.getToolNames(input); | |
t.deepEqual(toolNames, ["CodeQL command-line toolchain", "ESLint"]); | |
}); | |
ava_1.default('getMemoryFlag() should return the correct --ram flag', t => { | |
const totalMem = Math.floor(os.totalmem() / (1024 * 1024)); | |
const tests = { | |
"": `--ram=${totalMem - 256}`, | |
"512": "--ram=512", | |
}; | |
for (const [input, expectedFlag] of Object.entries(tests)) { | |
process.env['INPUT_RAM'] = input; | |
const flag = util.getMemoryFlag(); | |
t.deepEqual(flag, expectedFlag); | |
} | |
}); | |
ava_1.default('getMemoryFlag() throws if the ram input is < 0 or NaN', t => { | |
for (const input of ["-1", "hello!"]) { | |
process.env['INPUT_RAM'] = input; | |
t.throws(util.getMemoryFlag); | |
} | |
}); | |
ava_1.default('getThreadsFlag() should return the correct --threads flag', t => { | |
const numCpus = os.cpus().length; | |
const tests = { | |
"0": "--threads=0", | |
"1": "--threads=1", | |
[`${numCpus + 1}`]: `--threads=${numCpus}`, | |
[`${-numCpus - 1}`]: `--threads=${-numCpus}` | |
}; | |
for (const [input, expectedFlag] of Object.entries(tests)) { | |
process.env['INPUT_THREADS'] = input; | |
const flag = util.getThreadsFlag(); | |
t.deepEqual(flag, expectedFlag); | |
} | |
}); | |
ava_1.default('getThreadsFlag() throws if the threads input is not an integer', t => { | |
process.env['INPUT_THREADS'] = "hello!"; | |
t.throws(util.getThreadsFlag); | |
}); | |
ava_1.default('getRef() throws on the empty string', t => { | |
process.env["GITHUB_REF"] = ""; | |
t.throws(util.getRef); | |
}); | |
ava_1.default('isLocalRun() runs correctly', t => { | |
const origLocalRun = process.env.CODEQL_LOCAL_RUN; | |
process.env.CODEQL_LOCAL_RUN = ''; | |
t.assert(!util.isLocalRun()); | |
process.env.CODEQL_LOCAL_RUN = 'false'; | |
t.assert(!util.isLocalRun()); | |
process.env.CODEQL_LOCAL_RUN = '0'; | |
t.assert(!util.isLocalRun()); | |
process.env.CODEQL_LOCAL_RUN = 'true'; | |
t.assert(util.isLocalRun()); | |
process.env.CODEQL_LOCAL_RUN = 'hucairz'; | |
t.assert(util.isLocalRun()); | |
process.env.CODEQL_LOCAL_RUN = origLocalRun; | |
}); | |
ava_1.default('prepareEnvironment() when a local run', t => { | |
const origLocalRun = process.env.CODEQL_LOCAL_RUN; | |
process.env.CODEQL_LOCAL_RUN = 'false'; | |
process.env.GITHUB_JOB = 'YYY'; | |
util.prepareLocalRunEnvironment(); | |
// unchanged | |
t.deepEqual(process.env.GITHUB_JOB, 'YYY'); | |
process.env.CODEQL_LOCAL_RUN = 'true'; | |
util.prepareLocalRunEnvironment(); | |
// unchanged | |
t.deepEqual(process.env.GITHUB_JOB, 'YYY'); | |
process.env.GITHUB_JOB = ''; | |
util.prepareLocalRunEnvironment(); | |
// updated | |
t.deepEqual(process.env.GITHUB_JOB, 'UNKNOWN-JOB'); | |
process.env.CODEQL_LOCAL_RUN = origLocalRun; | |
}); | |
//# sourceMappingURL=util.test.js.map |