Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
codeql-action/node_modules/lodash/_basePullAt.js
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
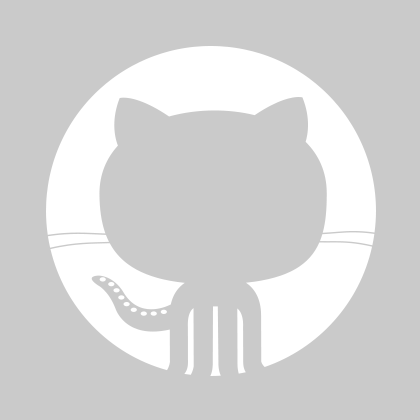
37 lines (32 sloc)
939 Bytes
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
var baseUnset = require('./_baseUnset'), | |
isIndex = require('./_isIndex'); | |
/** Used for built-in method references. */ | |
var arrayProto = Array.prototype; | |
/** Built-in value references. */ | |
var splice = arrayProto.splice; | |
/** | |
* The base implementation of `_.pullAt` without support for individual | |
* indexes or capturing the removed elements. | |
* | |
* @private | |
* @param {Array} array The array to modify. | |
* @param {number[]} indexes The indexes of elements to remove. | |
* @returns {Array} Returns `array`. | |
*/ | |
function basePullAt(array, indexes) { | |
var length = array ? indexes.length : 0, | |
lastIndex = length - 1; | |
while (length--) { | |
var index = indexes[length]; | |
if (length == lastIndex || index !== previous) { | |
var previous = index; | |
if (isIndex(index)) { | |
splice.call(array, index, 1); | |
} else { | |
baseUnset(array, index); | |
} | |
} | |
} | |
return array; | |
} | |
module.exports = basePullAt; |