Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
codeql-action/node_modules/lodash/_stackSet.js
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
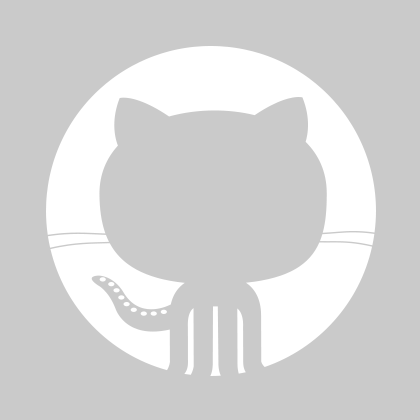
34 lines (31 sloc)
853 Bytes
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
var ListCache = require('./_ListCache'), | |
Map = require('./_Map'), | |
MapCache = require('./_MapCache'); | |
/** Used as the size to enable large array optimizations. */ | |
var LARGE_ARRAY_SIZE = 200; | |
/** | |
* Sets the stack `key` to `value`. | |
* | |
* @private | |
* @name set | |
* @memberOf Stack | |
* @param {string} key The key of the value to set. | |
* @param {*} value The value to set. | |
* @returns {Object} Returns the stack cache instance. | |
*/ | |
function stackSet(key, value) { | |
var data = this.__data__; | |
if (data instanceof ListCache) { | |
var pairs = data.__data__; | |
if (!Map || (pairs.length < LARGE_ARRAY_SIZE - 1)) { | |
pairs.push([key, value]); | |
this.size = ++data.size; | |
return this; | |
} | |
data = this.__data__ = new MapCache(pairs); | |
} | |
data.set(key, value); | |
this.size = data.size; | |
return this; | |
} | |
module.exports = stackSet; |