Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
codeql-action/node_modules/lodash/concat.js
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
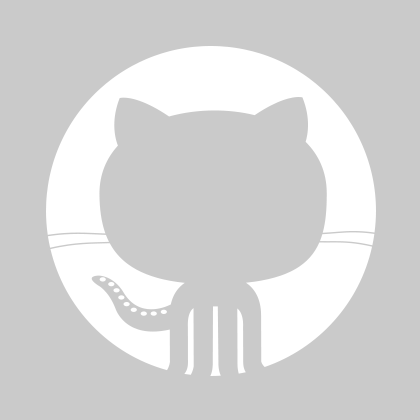
43 lines (40 sloc)
1007 Bytes
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
var arrayPush = require('./_arrayPush'), | |
baseFlatten = require('./_baseFlatten'), | |
copyArray = require('./_copyArray'), | |
isArray = require('./isArray'); | |
/** | |
* Creates a new array concatenating `array` with any additional arrays | |
* and/or values. | |
* | |
* @static | |
* @memberOf _ | |
* @since 4.0.0 | |
* @category Array | |
* @param {Array} array The array to concatenate. | |
* @param {...*} [values] The values to concatenate. | |
* @returns {Array} Returns the new concatenated array. | |
* @example | |
* | |
* var array = [1]; | |
* var other = _.concat(array, 2, [3], [[4]]); | |
* | |
* console.log(other); | |
* // => [1, 2, 3, [4]] | |
* | |
* console.log(array); | |
* // => [1] | |
*/ | |
function concat() { | |
var length = arguments.length; | |
if (!length) { | |
return []; | |
} | |
var args = Array(length - 1), | |
array = arguments[0], | |
index = length; | |
while (index--) { | |
args[index - 1] = arguments[index]; | |
} | |
return arrayPush(isArray(array) ? copyArray(array) : [array], baseFlatten(args, 1)); | |
} | |
module.exports = concat; |