Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
codeql-action/node_modules/lodash/create.js
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
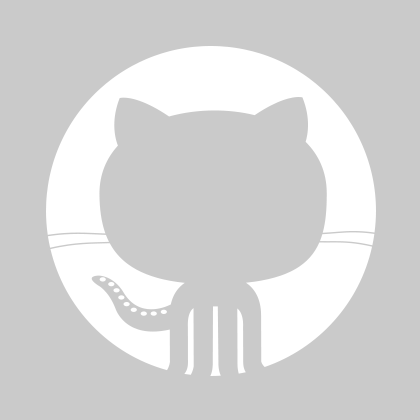
43 lines (41 sloc)
1.01 KB
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
var baseAssign = require('./_baseAssign'), | |
baseCreate = require('./_baseCreate'); | |
/** | |
* Creates an object that inherits from the `prototype` object. If a | |
* `properties` object is given, its own enumerable string keyed properties | |
* are assigned to the created object. | |
* | |
* @static | |
* @memberOf _ | |
* @since 2.3.0 | |
* @category Object | |
* @param {Object} prototype The object to inherit from. | |
* @param {Object} [properties] The properties to assign to the object. | |
* @returns {Object} Returns the new object. | |
* @example | |
* | |
* function Shape() { | |
* this.x = 0; | |
* this.y = 0; | |
* } | |
* | |
* function Circle() { | |
* Shape.call(this); | |
* } | |
* | |
* Circle.prototype = _.create(Shape.prototype, { | |
* 'constructor': Circle | |
* }); | |
* | |
* var circle = new Circle; | |
* circle instanceof Circle; | |
* // => true | |
* | |
* circle instanceof Shape; | |
* // => true | |
*/ | |
function create(prototype, properties) { | |
var result = baseCreate(prototype); | |
return properties == null ? result : baseAssign(result, properties); | |
} | |
module.exports = create; |