Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
codeql-action/node_modules/lodash/invert.js
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
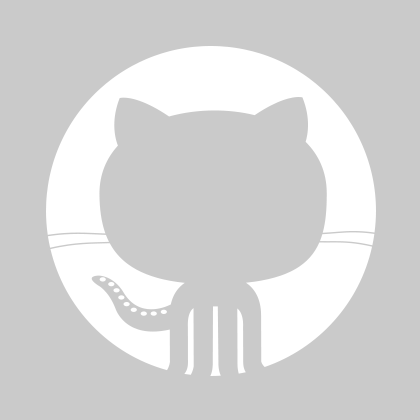
42 lines (37 sloc)
1.1 KB
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
var constant = require('./constant'), | |
createInverter = require('./_createInverter'), | |
identity = require('./identity'); | |
/** Used for built-in method references. */ | |
var objectProto = Object.prototype; | |
/** | |
* Used to resolve the | |
* [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring) | |
* of values. | |
*/ | |
var nativeObjectToString = objectProto.toString; | |
/** | |
* Creates an object composed of the inverted keys and values of `object`. | |
* If `object` contains duplicate values, subsequent values overwrite | |
* property assignments of previous values. | |
* | |
* @static | |
* @memberOf _ | |
* @since 0.7.0 | |
* @category Object | |
* @param {Object} object The object to invert. | |
* @returns {Object} Returns the new inverted object. | |
* @example | |
* | |
* var object = { 'a': 1, 'b': 2, 'c': 1 }; | |
* | |
* _.invert(object); | |
* // => { '1': 'c', '2': 'b' } | |
*/ | |
var invert = createInverter(function(result, value, key) { | |
if (value != null && | |
typeof value.toString != 'function') { | |
value = nativeObjectToString.call(value); | |
} | |
result[value] = key; | |
}, constant(identity)); | |
module.exports = invert; |