Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
codeql-action/node_modules/@octokit/plugin-retry/dist-node/index.js
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
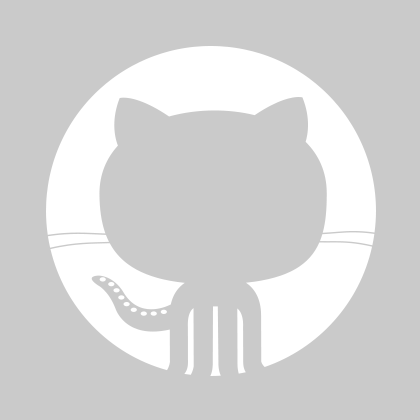
75 lines (59 sloc)
2.22 KB
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
'use strict'; | |
Object.defineProperty(exports, '__esModule', { value: true }); | |
function _interopDefault (ex) { return (ex && (typeof ex === 'object') && 'default' in ex) ? ex['default'] : ex; } | |
var Bottleneck = _interopDefault(require('bottleneck/light')); | |
// @ts-ignore | |
async function errorRequest(octokit, state, error, options) { | |
if (!error.request || !error.request.request) { | |
// address https://github.com/octokit/plugin-retry.js/issues/8 | |
throw error; | |
} // retry all >= 400 && not doNotRetry | |
if (error.status >= 400 && !state.doNotRetry.includes(error.status)) { | |
const retries = options.request.retries != null ? options.request.retries : state.retries; | |
const retryAfter = Math.pow((options.request.retryCount || 0) + 1, 2); | |
throw octokit.retry.retryRequest(error, retries, retryAfter); | |
} // Maybe eventually there will be more cases here | |
throw error; | |
} | |
// @ts-ignore | |
async function wrapRequest(state, request, options) { | |
const limiter = new Bottleneck(); // @ts-ignore | |
limiter.on("failed", function (error, info) { | |
const maxRetries = ~~error.request.request.retries; | |
const after = ~~error.request.request.retryAfter; | |
options.request.retryCount = info.retryCount + 1; | |
if (maxRetries > info.retryCount) { | |
// Returning a number instructs the limiter to retry | |
// the request after that number of milliseconds have passed | |
return after * state.retryAfterBaseValue; | |
} | |
}); | |
return limiter.schedule(request, options); | |
} | |
const VERSION = "3.0.3"; | |
function retry(octokit, octokitOptions = {}) { | |
const state = Object.assign({ | |
enabled: true, | |
retryAfterBaseValue: 1000, | |
doNotRetry: [400, 401, 403, 404, 422], | |
retries: 3 | |
}, octokitOptions.retry); | |
octokit.retry = { | |
retryRequest: (error, retries, retryAfter) => { | |
error.request.request = Object.assign({}, error.request.request, { | |
retries: retries, | |
retryAfter: retryAfter | |
}); | |
return error; | |
} | |
}; | |
if (!state.enabled) { | |
return; | |
} | |
octokit.hook.error("request", errorRequest.bind(null, octokit, state)); | |
octokit.hook.wrap("request", wrapRequest.bind(null, state)); | |
} | |
retry.VERSION = VERSION; | |
exports.VERSION = VERSION; | |
exports.retry = retry; | |
//# sourceMappingURL=index.js.map |