Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
codeql-action/node_modules/color-convert/index.js
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
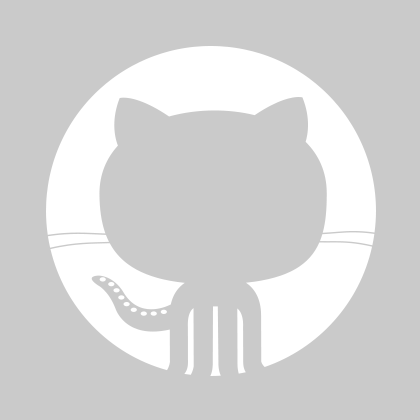
78 lines (58 sloc)
1.68 KB
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
var conversions = require('./conversions'); | |
var route = require('./route'); | |
var convert = {}; | |
var models = Object.keys(conversions); | |
function wrapRaw(fn) { | |
var wrappedFn = function (args) { | |
if (args === undefined || args === null) { | |
return args; | |
} | |
if (arguments.length > 1) { | |
args = Array.prototype.slice.call(arguments); | |
} | |
return fn(args); | |
}; | |
// preserve .conversion property if there is one | |
if ('conversion' in fn) { | |
wrappedFn.conversion = fn.conversion; | |
} | |
return wrappedFn; | |
} | |
function wrapRounded(fn) { | |
var wrappedFn = function (args) { | |
if (args === undefined || args === null) { | |
return args; | |
} | |
if (arguments.length > 1) { | |
args = Array.prototype.slice.call(arguments); | |
} | |
var result = fn(args); | |
// we're assuming the result is an array here. | |
// see notice in conversions.js; don't use box types | |
// in conversion functions. | |
if (typeof result === 'object') { | |
for (var len = result.length, i = 0; i < len; i++) { | |
result[i] = Math.round(result[i]); | |
} | |
} | |
return result; | |
}; | |
// preserve .conversion property if there is one | |
if ('conversion' in fn) { | |
wrappedFn.conversion = fn.conversion; | |
} | |
return wrappedFn; | |
} | |
models.forEach(function (fromModel) { | |
convert[fromModel] = {}; | |
Object.defineProperty(convert[fromModel], 'channels', {value: conversions[fromModel].channels}); | |
Object.defineProperty(convert[fromModel], 'labels', {value: conversions[fromModel].labels}); | |
var routes = route(fromModel); | |
var routeModels = Object.keys(routes); | |
routeModels.forEach(function (toModel) { | |
var fn = routes[toModel]; | |
convert[fromModel][toModel] = wrapRounded(fn); | |
convert[fromModel][toModel].raw = wrapRaw(fn); | |
}); | |
}); | |
module.exports = convert; |