Permalink
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
codeql-action/node_modules/crypt/crypt.js
Go to fileThis commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
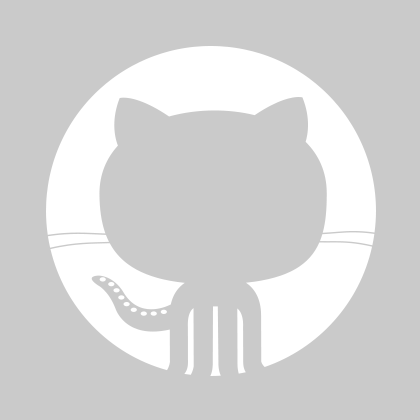
96 lines (83 sloc)
2.9 KB
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
(function() { | |
var base64map | |
= 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/', | |
crypt = { | |
// Bit-wise rotation left | |
rotl: function(n, b) { | |
return (n << b) | (n >>> (32 - b)); | |
}, | |
// Bit-wise rotation right | |
rotr: function(n, b) { | |
return (n << (32 - b)) | (n >>> b); | |
}, | |
// Swap big-endian to little-endian and vice versa | |
endian: function(n) { | |
// If number given, swap endian | |
if (n.constructor == Number) { | |
return crypt.rotl(n, 8) & 0x00FF00FF | crypt.rotl(n, 24) & 0xFF00FF00; | |
} | |
// Else, assume array and swap all items | |
for (var i = 0; i < n.length; i++) | |
n[i] = crypt.endian(n[i]); | |
return n; | |
}, | |
// Generate an array of any length of random bytes | |
randomBytes: function(n) { | |
for (var bytes = []; n > 0; n--) | |
bytes.push(Math.floor(Math.random() * 256)); | |
return bytes; | |
}, | |
// Convert a byte array to big-endian 32-bit words | |
bytesToWords: function(bytes) { | |
for (var words = [], i = 0, b = 0; i < bytes.length; i++, b += 8) | |
words[b >>> 5] |= bytes[i] << (24 - b % 32); | |
return words; | |
}, | |
// Convert big-endian 32-bit words to a byte array | |
wordsToBytes: function(words) { | |
for (var bytes = [], b = 0; b < words.length * 32; b += 8) | |
bytes.push((words[b >>> 5] >>> (24 - b % 32)) & 0xFF); | |
return bytes; | |
}, | |
// Convert a byte array to a hex string | |
bytesToHex: function(bytes) { | |
for (var hex = [], i = 0; i < bytes.length; i++) { | |
hex.push((bytes[i] >>> 4).toString(16)); | |
hex.push((bytes[i] & 0xF).toString(16)); | |
} | |
return hex.join(''); | |
}, | |
// Convert a hex string to a byte array | |
hexToBytes: function(hex) { | |
for (var bytes = [], c = 0; c < hex.length; c += 2) | |
bytes.push(parseInt(hex.substr(c, 2), 16)); | |
return bytes; | |
}, | |
// Convert a byte array to a base-64 string | |
bytesToBase64: function(bytes) { | |
for (var base64 = [], i = 0; i < bytes.length; i += 3) { | |
var triplet = (bytes[i] << 16) | (bytes[i + 1] << 8) | bytes[i + 2]; | |
for (var j = 0; j < 4; j++) | |
if (i * 8 + j * 6 <= bytes.length * 8) | |
base64.push(base64map.charAt((triplet >>> 6 * (3 - j)) & 0x3F)); | |
else | |
base64.push('='); | |
} | |
return base64.join(''); | |
}, | |
// Convert a base-64 string to a byte array | |
base64ToBytes: function(base64) { | |
// Remove non-base-64 characters | |
base64 = base64.replace(/[^A-Z0-9+\/]/ig, ''); | |
for (var bytes = [], i = 0, imod4 = 0; i < base64.length; | |
imod4 = ++i % 4) { | |
if (imod4 == 0) continue; | |
bytes.push(((base64map.indexOf(base64.charAt(i - 1)) | |
& (Math.pow(2, -2 * imod4 + 8) - 1)) << (imod4 * 2)) | |
| (base64map.indexOf(base64.charAt(i)) >>> (6 - imod4 * 2))); | |
} | |
return bytes; | |
} | |
}; | |
module.exports = crypt; | |
})(); |